RxJS beginner learning resources
Carefully curated list of best RxJS learning resources for beginners
RxJS is such a wonderful technology. Seriously. It's too bad that not many people outside Angular use it. But I totally get it. The entry bar is really high. Some say it's even higher than Vim's.
I learned Vim and I learned RxJS. I didn't say it was easy. Was it worth it? 100%. The key is being persistent and not give up. If I could learn so can you!
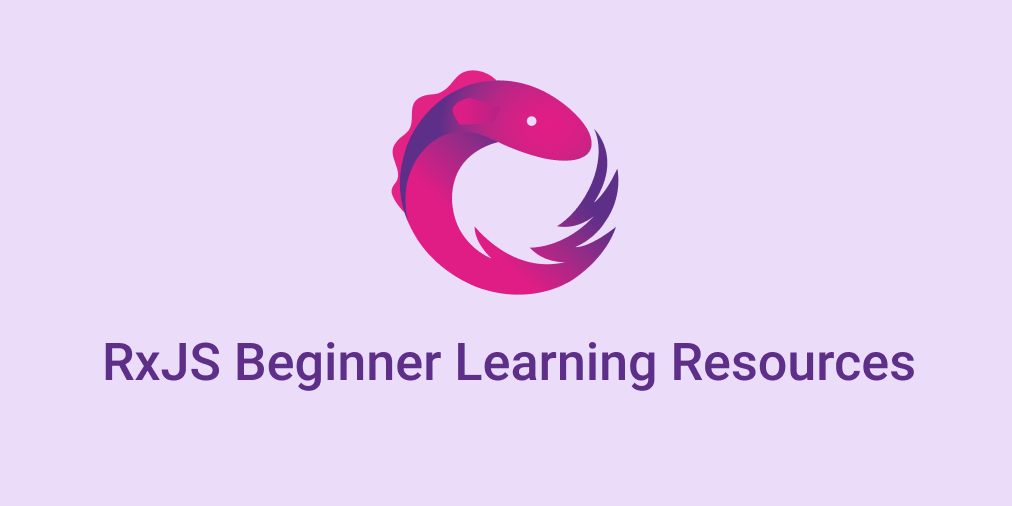
I've compiled a list of some of the best RxJS resources for people who are just getting started on their wonderful journey to become RxJS masters.
What is RxJS?#
Observables, multicast, share, subscriptions, hot, cold, async, streams, forkJoin, switchMap, concat. Oh my! Those terms alone can scare one off. But don't be afraid! Once you follow this guide everything will start making sense.
The way I like to think about RxJS is that it's a library that helps us process our data through a data-processing pipeline we define.
When you hear people talk about RxJS you often hear the word streams. Well, it kind of makes sense. The data flows through the processing pipeline just like water flows through a pipe.
RxJS also contains the word reactive in it. Let's talk about it and what reactivity means in this context. I like to think of it as the data pipeline only activates (reacts) only when we tell it to. What do I mean by that? Let me explain.
Reactivity means that we can trigger our data processing pipeline by various inputs or events that we define in the pipeline. But there is a catch. The pipeline is not activated, even when triggered by our defined events, unless we tell it that we are interested by subscribing to it. No subscribers, no reactivity, no data.
This works as a kind of pull model, even though the data is pushed through the pipeline. Let me explain. When we subscribe to our pipeline (pull), we activate the pipeline. It then activates the pipeline from the bottom all the way up by saying "Hey, we have a subscriber! Let's start working!" All the events listeners and triggers will get activated and they will start processing (pushing) data through our pipeline all the way down to us when something is triggered. When we unsubscribe, the pipeline will become inactive again, because there are no subscribers.
Does it make sense? It's reactive because it's lazy. Nothing happens until you subscribe. Only then it starts reacting (or working).
RxJS is also a declarative framework. This is another concept that can be hard to understand. With imperative (normal) programming you describe exactly what you want to happen, step by step. You give precise instructions. With declarative programming, on the other hand, you write code that describes what you want to do, but not necessarily how to do it. You say what you want, but you don't specify exactly how. It's compiler's job to figure it out. You give the compiler commands instead of telling it exactly what to do. These commands consists of RxJS operators chained together. The implementation details are abstracted away from you. You specify the desired outcome and compiler will figure it out for you.
This is good, because you are forced to work on the higher level of abstraction. The implementation details are already in place for you. Less code to write. You just have to define the desired outcome in your program and RxJS will take care of it.
Another hurdle, and maybe the hardest to jump over, is thinking in streams. Thinking in streams is super-hard and will take you some time to learn. But it's really cool once you grok it. When you finally reach that "Aha!" moment you will for sure get a rush. Suddenly, it will all make sense and you will realize how to merge, delay, filter, split and do lots of other cool things with streams.
One essential thing when learning RxJS is to take it slow and be patient. You head will hurt, for sure. Try not to get frustrated. It will all click together soon if you are persistent. Later, you will wonder why you didn't learn RxJS earlier and will be telling all your friends how cool it is and you will start prompting it in your circles.
How to use this guide#
I've compiled a list of resources that helped me learn RxJS. RxJS is built into Angular, that's why many articles on the internet are Angular based. But you can ignore all Angluar stuff and concentrate only on the juicy RxJS parts.
The guide is broken down into different sections. Start at the top, it will help you learn the core concepts. Later, feel free to jump around to the articles that catch your attention.
When you read an article and learn a new concept, it's not guaranteed to be crystal clear at first. Come back to it sometimes later and re-read it.
After you learn a new concept or operator, try to play around with it to get that hands-on feeling. Spend 20-30 minutes on it. It will be time well invested.
Also, many of the articles included are on Medium. Sorry about that.
Note on Svelte and RxJS
I am a Svelte fan, so I also threw in a couple of Svelte related articles for other Svelte fans out there. One thing about Svelte and RxJS combo is that they go very well together. I like to say that RxJS is Svelte's stores on steroids. You get 200% reactivity if you use RxJS.
Another cool thing is that you don't have to use onMount
, when fetching data for example. Why? Because RxJS is lazy (reactive). Since Svelte views RxJS pipelines as Svelte stores, Svelte compiler manages subscriptions for us automatically. Now, how cool is that?!
Basics#
The hardest part is getting started. RxJS has many operators, but you only need to learn a handful of them to be productive. Once you learn them, others will start making sense.
The introduction to Reactive Programming you've been missing
Classic introduction to Reactive Programming. This should be your starting point. It's a little dated, but explains the concepts well and teaches you how to think in streams.
Recreating a classic FRP tutorial with Svelte and RxJS
My own take on the tutorial above with Svelte and newer version of RxJS. Plus I went a bit further than the original article by removing code duplication.
Good and short introduction to RxJS in form of free online book. Highly recommended!
I explain why Svelte and RxJS is such a nice combo with a few simple examples.
Short article that gives a digestible overview of RxJS and concepts with a few code examples.
Thinking reactive with the SIP principle
Nice article that teaches you to think reactively with RxJS. I like to think of RxJS operators as Lego pieces that you assemble into something larger.
RxJS, where is the If-Else Operator
Coming from imperative background you might wonder where the if
statement is. Well, there is no if
statement sort of. Instead you use operators like map
and filter
to achieve the desired branching logic.
Understanding hot vs cold Observables
Hot and cold observables. You will hear it a lot when learning RxJS and will most likely get burned by it sometimes. This short article explains the concepts very well.
RxJS: Understanding the publish and share Operators
This article explains in-depth how to turn cold observarbles into hot. Heavy reading, but an excellent reference.
RxJS subjects is another concept that you must understand. They are really useful. This article explains subjects on the higher level.
RxJS Subjects Explained with Examples
Good code examples of RxJS subjects. A follow up article to the one above.
Comprehensive Guide to Higher-Order RxJs Mapping Operators
Mapping operators are the core of RxJS and there are quite a few of them. This article explains them well.
Understanding RxJS map, mergeMap, switchMap and concatMap
Another excellent article on various RxJS mapping operators. A must read!
RxJs Error Handling: Complete Practical Guide
You will get errors and exceptions when working with RxJS and you need to know how to handle them. This in-depth article explains how to deal with errors the RxJS way.
Little dated, but still very good RxJS concepts overview from Fireship. With complementary video too!
Intermediate#
Once you get down the basics your imperative mind will still struggle to translate it to declarative thinking. You need to revisit the concepts and examine them closer, more in-depth.
After you learn the basics, you only need to learn a handful of operators. Like, really learn them. Especially different mapping operators. Higher-order observables can be tough to grasp.
Below is a collection of intermediate resources. They all require basic RxJS knowledge. Without it they will be either overwhelming or will just not make any sense to you.
Thinking in nested streams with RxJS
Learn how to work with higher-order observables aka nested streams.
RxJS. Transformation Operators in Examples (part 1)
Very good breakdown of the transformation operators such as different buffer and concat operators. Clear code examples.
RxJS. Transformation Operators in Examples (part 2)
Second part of the transformation operators. This time various merge, scan, group and window operators. Excellent code examples!
Combining Observables with forkJoin in RxJS
ForkJoin is RxJS version of Promise.all
. It's really useful to have when you have to deal with parallel HTTP requests for example.
SwitchMap is one operator that you will use often. This is a nice breakdown of how it works using HTTP requests examples.
RxJS: merge() vs. mergeAll() vs. mergeMap()
Merge is also one of the frequently used operators. Make sure you understand all the different variations of it.
The magic of RXJS sharing operators and their differences
Explains the sharing operators in detail. Those cold vs hot observable concepts.
Creating Custom Operators in RxJS
Learn how to create custom observables in RxJS. Helps you understand and solidify your RxJS observable concepts.
Getting to Know the Defer Observable in RxJS
Defer operator is really handy. It might not be something you will use often, but it's still very important operator to know.
Create a tapOnce custom Rxjs Operator
Continuing on the topic of custom RxJS operators. Here is a very good article explaining how to create a custom tapOnce
operator. You will be using tap
operator a lot when you need to debug your pipelines and see what data flows through them.
Showing a loading spinner delayed with rxjs
Clean example of how to show a loading spinner while waiting for something. I am sure you will want to show a loading spinner in your apps.
Debounce with vanilla JavaScript or RxJS
Examples of debounce using plain JS and RxJS. Which one is better? You be the judge.
A text transcript and source code of the paid RxJS course on Egghead.io. Covers lots of ground!
Advanced#
Below are some advanced topics and tips. Take a look at them when you are really sure you understand the core concepts.
Learn the expand
operator with guitar delay pedal example. Very cool!
RxJS examples of the day (part 1)
Good examples of how solve problems in the most effective ways when using RxJS. Lots of learning opportunities.
RxJS: iif is not the same as defer with ternary operator
Comparison between iif
and defer
and when to use what.
Building a safe autocomplete operator in RxJS
RxJS is really handy for autocomplete. Learn how to build a custom autocomplete operator.
Thinking reactively in Angular and RXJS
Learn how to think reactively by building a calendar app.
Other Interesting Resources#
Best RxJS reference with good examples. My goto place when I need to look up an operator.
Fun game to learn RxJS. You have to code your way through.
RxJS is usually explained with the help of marble diagrams. This is a good interactive reference to many RxJS operators.
Another interactive tool slash reference that will help you understand operators with code examples and marble diagrams.
Lots of good resources and short interactive animations comparing different operators together. Site feels a bit messy, but the information is really good.
Firebase has really nice RxJS bindings. This article explains the basics.
Introducing BLoC Pattern with React and RxJS
BLoC pattern originated in Dart language, but can be used in other frameworks too. Here is a simple example with RxJS in React.
RxJS Cheatsheet VS Code extension
Handy VSCode extension. View RxJS operator documentation in-place.
https://github.com/AlexAegis/svelte-minesweeper
Cool classic Minesweeper clone built in Svelte, RxJS and TypeScript. Lots of learning opportunities by studying the code.
Videos#
If videos are your thing, here are some of the best ones.
Essential talk that explains observables by building observables. If you plan on watching one talk only, make it this one.
Learn RxJS in 60 Minutes for Beginners
A nice crash course that covers the basics of RxJS. Covers lots of ground.
RxJS Recipes - Uphill Conf 2019
Sweet and short talk on how to solve a problem using RxJS. Learn how to think in streams and patterns. Required watching.
Mastering the Subject: Communication Options in RxJS | Dan Wahlin
A really good explanation of RxJS subjects and how you can use them to communicate between different application components.
The Magic of RxJS - Natalia Tepluhina | JSHeroes 2019
Shows how you can use RxJS to build a Pong game. Heavy code, but very inspirational talk!
Data Composition with RxJS | Deborah Kurata
Really good talk that explains how you can use RxJS to fetch data and do cross-component communication. A must watch!
Thinking Reactively: Most Difficult | Mike Pearson
Learn how to think reactively by building a typeahead search. Very good talk for RxJS newbies.
Understanding RxJS Error Handling
Excellent talk on different exception handling strategies in RxJS.
Why Should You Care About RxJS Higher-order Mapping Operators?
Explains higher-order RxJS mapping operators with clear examples that all can understand.
I switched a map and you'll never guess what happened next
Fun interactive talk that explains mapping operators with the help of hiring agency example.
RxJS Advanced Patterns – Operate Heavily Dynamic UI’s | Michael Hladky
Advanced concepts talk that goes very deep. Expect to scrub a lot in order to understand everything.
Personal tips#
Here are some tips from me that can help you on your RxJS learning journey.
- Don't give up! If will be hard, but if you give it time, it will all come together soon. Things will click, I promise!
- Use
tap
operator for debugging your pipelines. Injecttap(console.log)
anywhere in your pipeline to view the data flowing through it. - You will probably start by composing very large pipelines. It's OK. Later, try to write small single-purpose operators instead and learn how to combine them. View them as Lego bricks.
- Learn
of
,from
,merge
,BehaviourSubject
,combineLatest
,startWith
and all the mapping operators. They will give you a solid base to stand on.
Exercises#
You can read articles and watch videos all you want, but in order to truly learn and understand you have to do some coding. Here are some good problems you can try to tackle with RxJS.
Timer App
Try to create a simple timer app. You should be able to start, stop and reset the timer. Bonus points: be able to set a countdown time.
Typeahead Search
Try to implement a simple typeahead TV show search by using Episodate API.
Closing words#
RxJS is a wonderful technology, but it's not widely adopted yet. One of the main reasons might be the lack of good learning resources. Hopefully this resource will help your discover its super-powers.
My main advice is to take it slow and don't give up! It will all click soon enough if you are persistent. Soon you will be wondering how you even could do something without RxJS and will mutter for yourself how easy you could have solved the problem if only this project used RxJS.